ConfinedOrbital¶
- class ConfinedOrbital(principal_quantum_number=None, angular_momentum=None, radial_cutoff_radius=None, confinement_start_radius=None, additional_charge=None, confinement_strength=None, confinement_power=None, radial_step_size=None)¶
Class for representing a confined atomic orbital.
- Parameters:
principal_quantum_number (int) – The all electron principal quantum number (n) of the orbital.
angular_momentum (int) – The angular momentum quantum Number (l) of the orbital.
radial_cutoff_radius (PhysicalQuantity of type length) – The distance from the core where the basis orbital is zero (compact support radius).
confinement_start_radius (PhysicalQuantity of type length) – Radial distance to where the confinement potential starts. The
confinement_start_radius
must be less than or equal to theradial_cutoff_radius
.additional_charge (float) – Charge of the atom when generating the basis function. Default:
0.0
confinement_strength (PhysicalQuantity of type energy) – The confinement strength of the potential confining the atomic orbital. Default:
20 * Hartree
confinement_power (int) – The confinement power for the potential. Default:
1
radial_step_size (PhysicalQuantity of type length) – The radial step size determining the distance between grid points on the linear radial grid. Default:
0.01 * Bohr
- additionalCharge()¶
- Returns:
The additional charge used for generating this
ConfinedOrbital
instance.- Return type:
float
- angularMomentum()¶
- Returns:
The angular momentum.
- Return type:
int
- confinementPower()¶
- Returns:
The power of the confinement potential.
- Return type:
int
- confinementStartRadius()¶
- Returns:
The radius where the confinement potential starts.
- Return type:
PhysicalQuantity of type length
- confinementStrength()¶
- Returns:
The strength of the confinement potential.
- Return type:
PhysicalQuantity of type energy
- nlinfo()¶
- Returns:
The nlinfo.
- Return type:
dict
- principalQuantumNumber()¶
- Returns:
The principal quantum number.
- Return type:
int
- radialCutoffRadius()¶
- Returns:
The radial cutoff radius.
- Return type:
PhysicalQuantity of type length
- radialStepSize()¶
- Returns:
The radial grid spacing.
- Return type:
PhysicalQuantity of type length
- uniqueString()¶
Return a unique string representing the state of the object.
Usage Examples¶
Define a BasisSet for Hydrogen:
hydrogen_1s = ConfinedOrbital(
principal_quantum_number = 1,
angular_momentum = 0,
radial_cutoff_radius = 5.28603678847*Bohr,
confinement_start_radius = 0.8 * 5.28603678847*Bohr,
additional_charge = 0.0,
confinement_strength = 20.000*Hartree,
confinement_power = 1,
radial_step_size = 0.001*Bohr,
)
my_hydrogen_basis = BasisSet(
element = Hydrogen,
orbitals = [hydrogen_1s],
occupations = [1.0],
pseudopotential = NormConservingPseudoPotential('normconserving/H.LDAPZ.zip'),
)
Notes¶
The basis functions are found by solving the radial Schrödinger equation for the atom with a confinement potential \(V_{\text{c}}(r)\). The confinement potential is defined by the parameters confinement_strength
(\(V_0\)), confinement_power
(\(n\)), confinement_start_radius
(\(r_i\)), and radial_cutoff_radius
(\(r_c\) ) through the equation
Fig. 148 shows the confinement potential and the corresponding basis functions used for constructing the LDA standard basis set for hydrogen.
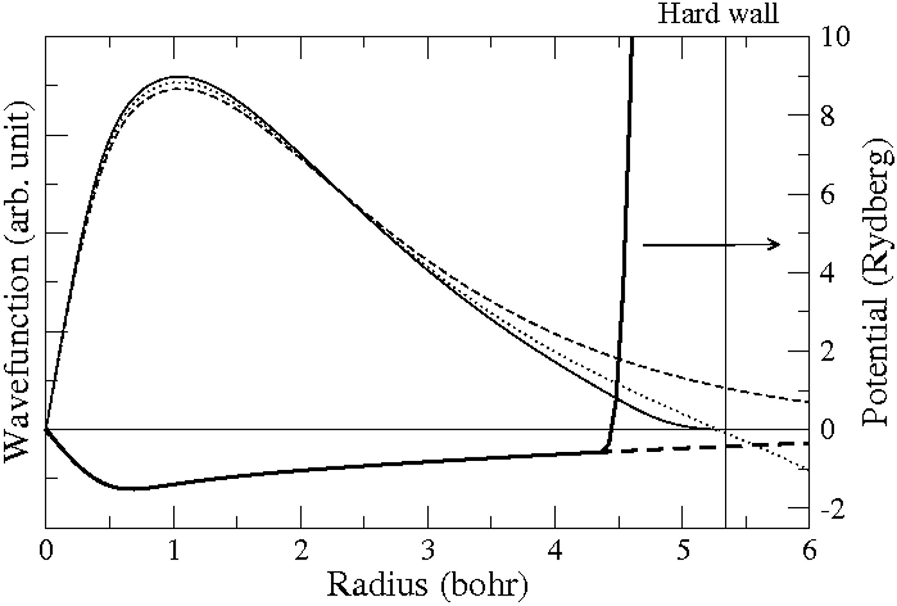
Fig. 148 The lower part of the plot shows the \(\ell=0\) effective potential for hydrogen (dashed) with the soft confinement potential (solid). The upper part shows the lowest occupied eigenstate of the confined potential (solid line), and the atomic s-wave function is indicated by the dashed curve (note that \(r\cdot \psi(r)\) are plotted).The dotted curve shows the radial wave function with energy 0.01 Ry above the atomic eigenenergy. The position of the first node of this solution defines the position of \(r_c\) .¶
For backwards compatibility, if \(V_0\) is given in units of energy\(\times\)length (as was the convention in QuantumATK 12.2 and earlier, where a slightly different expression was used for the confinement potential), the rescaled value \(V_0/(r_c-r_i)\) is used for the confinement strength, to conform to the new formula given above. Further information about the basis functions can be found in LCAO basis set.