genericAlloy¶
- genericAlloy(configurations, percentages, algorithm, repetitions, rng=None)¶
Create alloy by creating a super cell and mixing the given configurations.
- Parameters:
configurations (List of BulkConfigurations) – The configurations to use in the alloy.
percentages (List of floats) – The percentages.
algorithm (FixedFraction | NormalDistribution) – The algorithm to use.
repetitions (tuple (length 3)) – The repetitions in the A, B, and C directions.
rng (numpy.random.RandomState) – The random number generator used to generate the random number stream. If None, a new random number generator stream will be made with a seed obtained from the operating system’s entropy pool. Default: numpy.random.RandomState().
- Returns:
The created alloy.
- Return type:
Usage Examples¶
Generate a AuAg alloy with 50/50 composition and repeat the alloy 4x in all dimensions.
# -- Setup initial crystal configuration ---
# Set up lattice
lattice = FaceCenteredCubic(4.07825*Angstrom)
# Define elements
elements = [Gold]
# Define coordinates
fractional_coordinates = [[ 0., 0., 0.]]
# Set up configuration
Au_configuration = BulkConfiguration(
bravais_lattice=lattice,
elements=elements,
fractional_coordinates=fractional_coordinates
)
# Set up lattice
lattice = FaceCenteredCubic(4.0857*Angstrom)
# Define elements
elements = [Silver]
# Define coordinates
fractional_coordinates = [[ 0., 0., 0.]]
# Set up configuration
Ag_configuration = BulkConfiguration(
bravais_lattice=lattice,
elements=elements,
fractional_coordinates=fractional_coordinates
)
# --- Create an alloy ---
alloy_configuration = genericAlloy(
configurations=[Au_configuration, Ag_configuration],
percentages=[50.0, 50.0],
algorithm=Normal_distribution,
repetitions=(4, 4, 4)
)
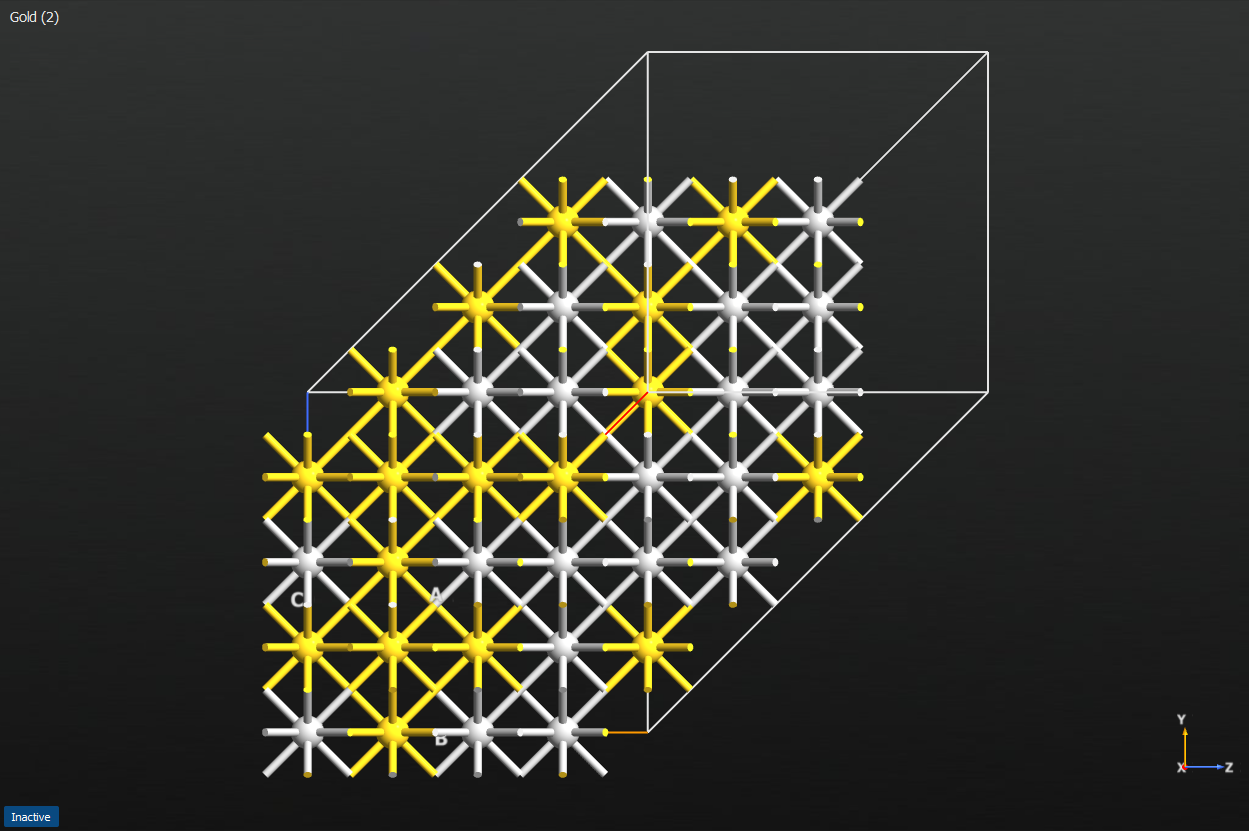
Fig. 184 Example alloy generated by genericAlloy. Yellow: Au, Grey/White: Ag. Note that the atom distribution is random each time genericAlloy is run.¶
Notes¶
The percentages must sum up to exactly 100.0.