changeDeviceLength¶
- changeDeviceLength(device_configuration, length, extreme)¶
Method to alter the device length. Handles devices with transverse electrode repetitions.
- Parameters:
device_configuration (
DeviceConfiguration
|SurfaceConfiguration
) – The configuration to shrink.length (PhysicalQuantity of type length) – The length by which the central region should be altered.
extreme (Left | Right) – Which side of the configuration to alter.
- Returns:
The altered device configuration.
- Return type:
Usage Examples¶
Change the device length to the left by 2.0391 Ang.
# --- Create input configuration ---
# Set up lattice
vector_a = [4.07825, 0.0, 0.0] * Angstrom
vector_b = [0.0, 4.07825, 0.0] * Angstrom
vector_c = [0.0, 0.0, 32.626] * Angstrom
lattice = UnitCell(vector_a, vector_b, vector_c)
# Define elements
elements = [Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold,
Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold,
Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold, Gold]
# Define coordinates
fractional_coordinates = [[ 0. , 0. , 0. ],
[ 0. , 0. , 0.125 ],
[ 0. , 0. , 0.25 ],
[ 0. , 0. , 0.375 ],
[ 0. , 0. , 0.5 ],
[ 0. , 0. , 0.625 ],
[ 0. , 0. , 0.75 ],
[ 0. , 0. , 0.875 ],
[ 0.5 , 0.5 , 0. ],
[ 0.5 , 0.5 , 0.125 ],
[ 0.5 , 0.5 , 0.25 ],
[ 0.5 , 0.5 , 0.375 ],
[ 0.5 , 0.5 , 0.5 ],
[ 0.5 , 0.5 , 0.625 ],
[ 0.5 , 0.5 , 0.75 ],
[ 0.5 , 0.5 , 0.875 ],
[ 0.5 , 0. , 0.0625],
[ 0.5 , 0. , 0.1875],
[ 0.5 , 0. , 0.3125],
[ 0.5 , 0. , 0.4375],
[ 0.5 , 0. , 0.5625],
[ 0.5 , 0. , 0.6875],
[ 0.5 , 0. , 0.8125],
[ 0.5 , 0. , 0.9375],
[ 0. , 0.5 , 0.0625],
[ 0. , 0.5 , 0.1875],
[ 0. , 0.5 , 0.3125],
[ 0. , 0.5 , 0.4375],
[ 0. , 0.5 , 0.5625],
[ 0. , 0.5 , 0.6875],
[ 0. , 0.5 , 0.8125],
[ 0. , 0.5 , 0.9375]]
# Set up configuration
bulk_configuration = BulkConfiguration(
bravais_lattice=lattice,
elements=elements,
fractional_coordinates=fractional_coordinates
)
# Create a basic device configuration.
configuration = deviceFromBulk(
bulk_configuration=bulk_configuration,
electrode_extension_lengths=Automatic,
adjust_cell=True,
use_minimal_electrodes=All
)
# --- Change the device length ---
# Apply the desired extension/retraction to the left side of the central region.
configuration = changeDeviceLength(
device_configuration=configuration,
length=2.0391 * Angstrom,
extreme=Left
)
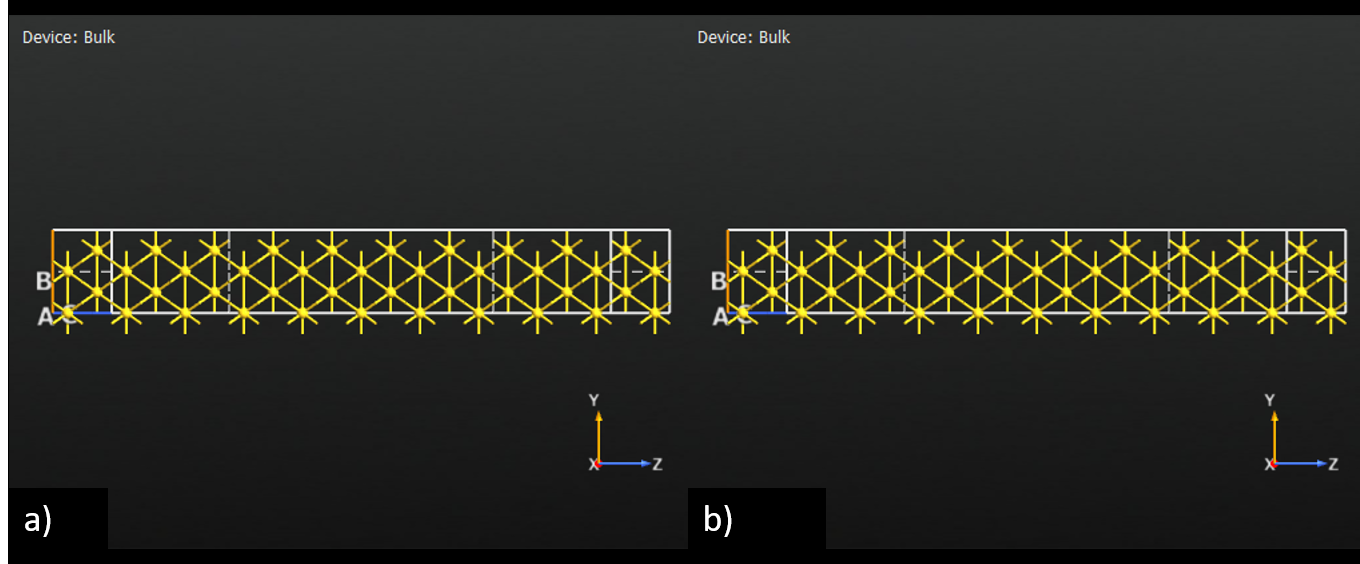
Fig. 178 a) The input configuration used in the above example. b) The DeviceConfiguration generated from the example.¶